Vue js Array Splice function: In Vue.js, the splice() method is used to add or remove elements from an array or array-like object. This method modifies the original array and returns the removed items as a new array. To use the splice() method in Vue.js, you first need to get a reference to the array using data binding. You can then call the splice() method on this array and pass in the starting index, number of items to remove, and any items to add. This can be useful for dynamically updating lists or tables in your Vue.js application. Overall, the splice() method provides a powerful tool for manipulating arrays in Vue.js.
How does the Vue.js splice() array function work and can you provide an example of how to use it to add or delete items from an array in Vue.js?
Vue JS is a JavaScript framework that simplifies the process of building user interfaces. Adding or removing elements from a JavaScript array in Vue JS is straightforward. You can use the splice()
method to add or remove elements from an array. To add an item to the array, you can use the splice()
method with the index where you want to add the new item and a zero value for the deleteCount parameter. To remove an item from the array, you can use the splice()
method with the index where you want to remove the item and a deleteCount of one. With Vue JS, you can easily manipulate arrays and update the user interface accordingly.
Vue Js Add/Remove elements/item from array Example
<div id="app">
<button @click="addFun">Add </button>
<button @click="delFun">Delete</button>
<p>{{demoArray}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
demoArray : ['Vue','React','Angular','Node','Php']
}
},
methods:{
addFun(){
this.demoArray.splice(3,0,'SQL');
},
delFun(){
this.demoArray.splice(3,1)
}
}
}).mount('#app')
</script>
The output of above example
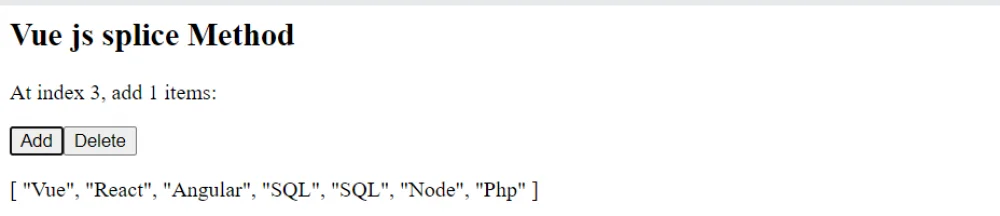
What is an example of removing 3 elements from index 1 in Vue.js?
To remove 3 elements from index 1 in Vue.js, you can use the splice
method on the array. The splice
method changes the content of an array by removing or replacing elements. To use it, you need to pass the starting index, the number of elements to remove, and optionally, the new elements to add
Vue Js Remove items | Example
<div id="app">
<button @click="delFun">Delete</button>
<p>{{demoArray}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
demoArray : ['Vue','React','Angular','Node','Php']
}
},
methods:{
delFun(){
this.demoArray.splice(1,3)
}
}
}).mount('#app')
</script>
This code removes three elements starting from index 1 in the array
variable. After the code runs, the array will have three fewer elements, and all the remaining elements will be shifted down to fill the gap.
Output of above example
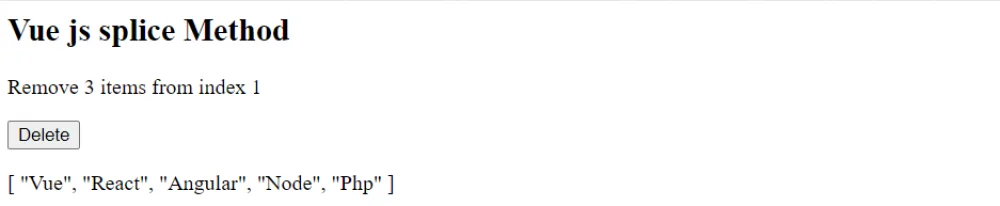
How can you use the slice method in Vue.js to add a new element to the last index of an array?
The “createApp” method is called with an object as an argument, which specifies the application’s data, methods, and other options. In the “data” object, the “demoArray” property is an array of five strings, representing different web development frameworks and programming languages.
The “methods” object contains the “addFun” method, which is triggered when the “Add” button is clicked. This method uses the “splice” method to add a new string “SQL” at index 5 (i.e., after the last item in the array).
Vue js Add items | Example
<div id="app">
<button @click="addFun">Add </button>
<p>{{demoArray}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
demoArray: ['Vue','React','Angular','Node','Php']
}
},
methods:{
addFun(){
this.demoArray.splice(5,0,'SQL');
},
}
}).mount('#app')
</script>
Output of above example
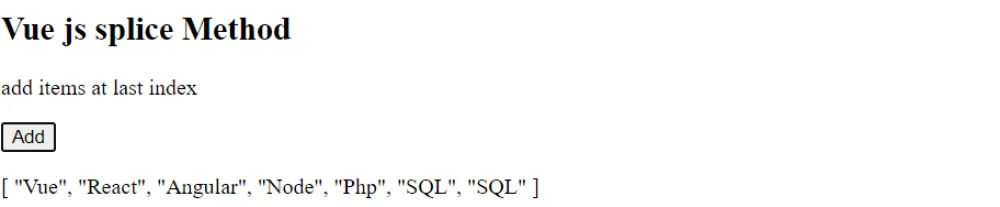
What is the best way to eliminate all elements in a list after the 4th index?
The splice method in Vue JS is used to modify an array by removing or adding elements. In this example, we are using splice to remove all the elements that come after a specified index in the array. By passing the index to the splice method, we can remove all the elements that come after it, effectively truncating the array. This can be useful in situations where we only need to keep a certain number of elements in the array, or when we want to remove unnecessary data
Vue Js remove all item from array by index | Example
<div id="app">
<button @click="addFun">Remove all</button>
<p>{{language}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
language : ['Vue','React','Angular','Node','Php','SQL','Express']
}
},
methods:{
addFun(){
this.language.splice(4);
},
}
}).mount('#app')
</script>
Output of above example

How can I remove an object from an array using JavaScript in a Vue.js application?”
In Vue.js, you can remove an item from an array by using the JavaScript splice() method. The splice() method takes two parameters: the starting index and the number of items to remove.
In the example given, you can remove the object with s.no. = 4 by finding its index in the array, and then passing that index and the number 1 (since we only want to remove one item) as arguments to the splice() method.
Delete object property Vue JS
<div id="app">
<button @click="addFun">Remove</button>
<table>
<tr>
<th>S.no</th>
<th>Site Name</th>
<th>Site Url</th>
</tr>
<tr v-for="object in demoObject">
<td> {{object.s_no}}</td>
<td>{{object.siteName}}</td>
<td><a :href="object.siteUrl" target="_blank">{{object.siteUrl}}</a></td>
</tr>
</table>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
demoObject : [
{
s_no:1,
siteName:'Sarkari Naukri Exams',
siteUrl:'https://sarkarinaukriexams.com'
},
{
s_no:2,
siteName:'Font Awesome Icons',
siteUrl:'https://fontawesomeicons.com'
},
{
s_no:3,
siteName:'Tutorials Plane',
siteUrl:'https://tutorialsplane.com'
},
{
s_no:4,
siteName : 'Schools Geek Technology',
siteUrl:'https://schoolsgeek.com'
}
]
}
},
methods:{
addFun(){
this.demoObject.splice(2,1);
},
}
}).mount('#app')
</script>
Output of above example
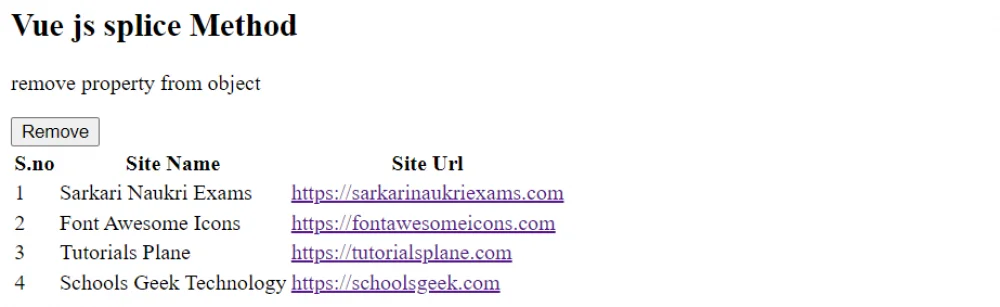